|
本帖最后由 math_comix 于 2014-1-8 10:01 编辑
原帖地址: http://www.lejos.org/forum/viewtopic.php?f=18&t=5359 By Aswin
英文好的就直接看原文吧,楼主英文战5渣,意译为主,错了表打我
LeJOS 0.5.0-alpha introduces a uniform framework for sensors. This post explains some of the concepts of the framework and provides some examples as well.
对于各种传感器,LejOS 0.5.0-alpha 引入了统一的框架。本帖介绍一些基本概念并且附上一些实例。
Naming
Sensor classes now follow a strict naming convention. This should help you to quickly find the right class for your sensor.
The first part of the class name is EV3 for EV3 sensors made by Lego. NXT for Lego sensors made for the NXT or the name of the manufacturer. The second part consists of the full sensor name (as used by the manufacturer). There might by a third part to the class name, a V followed by a version number. It is used to distinguish between hardware versions of a sensor. For example, the ultrasonic sensor that ships with the EV3 is called EV3UltrasonicSensor, the angle sensor from HiTechnic is called HiTechnicAngleSensor.
All sensor classes are found in the lejos.hardware.sensor package.
Sensor类现在有了严格的命名规定。这将帮助你快速找到对应的类。
对于EV3版的传感器,类名称的第一部分是EV3;以NXT开头的类,用于NXT的传感器;还有一些以制造商名字开头的类。
类名称的第二部分是传感器的全称。
有些类的名字还有第三部分,就是以V开头的版本号,主要是用来区分传感器的硬件版本。
举例:EV3版的超声波传感器对应的类叫做EV3UltrasonicSensor,HiTechnic公司生产的角度传感器叫做HiTechnicAngleSensor
所有的传感器类都可以在lejos.hardware.sensor找到。
Samples
Sensor make measurements, in LeJOS a measurement is called a sample. A sample consists of one ore more values taken at one moment in time. A sound sensor for example returns one value at a time, some accelerometers return three values at a time. Mo matter how much values a sensor measures, they are in all cases returned in an array of floats. All sensors use the same method for getting a measurement: void fetchSample( float[] sample, int offset). The sensors also have a method to query the number of elements in a sample: int getSampleSize(). This number does not change over time. The methods are defined in the SampleProvider interface.
传感器产生度量(这句话真心不知道怎么翻译 ,大概意思大家都懂吧,类似于超声波传感器测量出距离),在LeJOS中,度量被称作 样本(sample)。一个样本(sample)包含了一个或多个数值。大家喜闻乐见的举例时间!一个Sound声音传感器每次采集只返回一个数值,许多Accelerometer加速度传感器每次返回3个数值(我猜是对应了X,Y,Z三个方向吧?没用过不了解)。无论传感器每次测量出多少数值,这些数值都通过一个浮点型的数组返回。所有的传感器使用同样的方法获得一个度量:void fetchSample(float[] sample, int offset)。另外还有一个方法可以查询sample中包含几个元素:int getSampleSize(),这个size不会随时间改变。
以上方法在SampleProvider接口中定义。
Modes
Some sensors have multiple ways of operation. These are called modes. The EV3 ultrasonic sensor has both a mode to measure distance and a mode to listen to other ultrasonic sensors. LeJOS implements each mode as a class. An object for using a sensor in a particular mode can be obtained from the sensor class. There is no need for configuring the sensor for a particular mode, this is done internally by LeJOS. You can use different modes in one program. To do so you just need to obtain an object for each mode you want to use. You do not have to switch between modes, you just use the mode object of your choice. If needed the sensor is configured internally. This might take some time and you should be aware of that when mixing up different modes.
A sensor class that support multiple modes implements the SensorModes interface. It has a method to get an list of supported modes: ArrayList<String> getAvailableModes(). It also has a method to obtain a certain mode: SampleProvider getMode(int index) or: SampleProvider getMode(string modeName).
有一些传感器有多种操作方式。我们称之为模式modes。比如EV3的超声波传感器有两种模式:测距,监听其他超声波传感器。
lejos通过两个类分别实现其功能。无须设定传感器的模式mode,这事儿由LeJOS内部搞定。
你也可以在同一个程序里用不同的模式。咋做咧?你只需 对不同的模式mode 分别获得一个对象object。然后你不需要转换模式,想用什么模式就用什么模式的对象。
如果传感器的模式由lejos内部设定,这可能会需要一些时间,你需要在混合不同模式的时候加以注意。
如果一个传感器类支持多种模式,那么它就会有一个接口叫做SensorModes,其中有一个方法去获得一个列表list包含所支持的所有模式:ArrayList<String> getAvailableModes()。此外,这个类还有一个方法,可以获得特定的模式:SampleProvider getMode(int index)或者SampleProvider getMode(string modeName)
An example
The following example shows how to work with sensors that support multiple modes.
下面的例子说明了如何使用支持多种模式的传感器
// get a port instance 取得端口实例
Port port = LocalEV3.get().getPort("S2");
// Get an instance of the Ultrasonic EV3 sensor 取得EV3超声波传感器实例
SensorModes sensor = new EV3UltrasonicSensor(port);
// get an instance of this sensor in measurement mode 取得模式实例
SampleProvider distance= sensor.getModeName("Distance");
// initialise an array of floats for fetching samples 初始化sample数组
float[] sample = new float[distance.sampleSize()];
// fetch a sample 获得一个sample
distance.fetchSample(sample, 0);
Standard units
In LeJOS each sensor uses standard units. This makes sensors of different manifacturers interchangeable. It also simplifies further processing of a sample. For units LeJOS uses SI-units. So, distances are always returned in meters, acceleration is always returned in m/s^2, etc. Angles are always measured in degrees.
在LeJOS中,每一个传感器都使用标准单位。这使得不同厂商生产的传感器可以互相替换。这也简化了对于sample的进一步处理。LeJOS使用SI-units作为单位。因此,距离总是以米为单位,加速度以 m/s^2 为单位,以此类推。而角度以 度 作为单位。
Coordinate system
LeJOS uses a cartesian system. The positive X-axis points in the same direction as the plug you plug into a sensor. This is also the direction the Ultrasonic sensors from Lego point to. The positive Y-axis points to the left of the X-axis and the positive Z-axis points upwards. Angles follow the right hand rule. This means that a counter clockwise rotation of a robot is measured as a positive rotation by the sensors on it.
On sensors that support multiple axes, like some gyroscopes and accelerometers, the axis order in a sample is alway X,Y,Z.
LeJOS使用笛卡尔系统(其实就是我们常说的三围直角坐标系,见下图)。x轴的正半轴与你插入传感器的插头同向(这句话我不确定……请各位指正)。同时这也是乐高生产的超声波传感器指向的方向。y轴的正半轴指向x轴的左侧,z轴的正半轴指向上方。角度符合右手定则,这意味着,机器人逆时针转动时,其传感器采集到的是正方向转动。
对于那些支持多轴的传感器,比如一些陀螺仪gyroscope和加速度计accelerometer,sample中各轴的顺序总是X,Y,Z(sample[0]代表x轴读数,sample[1]代表y轴,sample[2]代表Z轴)
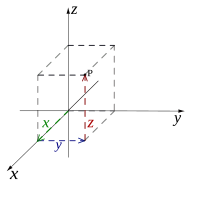
(翻译好辛苦 ,楼主开始怀疑自己的英文和中文是不是体育老师教的了……
Filters
Filters are used to alter a sample or , sometimes, to alter the flow of samples. They are an integral part of the framewok. Filters take a sample from a SampleProvider, modify it and then pass it on as a sample. They are in fact, sample providers themselves. LeJOS comes with some ready made filters, they are found in the lejos.robotics.filter package. The example below shows how to use a filter to get the running average of the last five samples of an ultrasonic sensor.As the code shows a filter constructor takes a sample provider as its first parameter followed by some configuration parameters. Instead of fetching a sample from the sensor class it is now fetched from the filter.
Filters can be stacked on top of each other to allow for more complex manipulations. The second filter takes the identifier of the first filter in its constructor and so on. One fetches the sample from the last filter in the stack.
Filters(这个词我就不翻译了,因为找不到准确的名称,总不能叫过滤器吧?)被用来改变一个sample或者某些时候改变一个sample流。
filter也是这个框架的一部分。filer通过sampleProvider取样,修改它再传给sample。实际上,它们就是sample provider本身。
LeJOS包含了一些现成的filter,它们在lejos.robotics.filter这个package中。
下面的例子展示了如何使用filter来获得一个平均值——最近五次超声波传感器取样的平均值。
正如代码所示,一个filter构造函数(constructor),第一个参数是一个sample provider,随后是一些设定参数。
不同于以往直接从传感器类获得样本sample,在这段代码里,sample通过filter获得。
filter可以被复合使用从而完成一些复杂的操作。例如,第二个filter将第一个filter的identifier作为构造函数的参数并且以此类推。一个filter获得通过上一个filter获得sample。
Port port = LocalEV3.get().getPort("S2");
// Get an instance of the Ultrasonic EV3 sensor
SensorModes sensor = new EV3UltrasonicSensor(port);
// get an instance of this sensor in measurement mode
SampleProvider distance= sensor.getModeName("Distance");
// stack a filter on the sensor that gives the running average of the last 5 samples
SampleProvider average = new MeanFilter(distance, 5);
// initialise an array of floats for fetching samples
float[] sample = new float[average.sampleSize()];
// fetch a sample
average.fetchSample(sample, 0);
Backward compatibility
LeJOS 0.5.0-alpha deals with sensors in a different way than its predecessors. In general it does not provide backward compatibility. There are a few exceptions though. There are some adaptors in the lejos.robotics package that do provide backward compatibility. If you do not want to alter your existing programs too much, it is advised to look in this package for an adaptor that makes a sensor backward compatible. An adaptor can be stacked on a sensor of filter just like a filter. If there is no adaptor aailable for your sensor, then the available adaptors in the package provide a good example of writing an adaptor youself.
LeJOS 0.5.0-alpha采用了与之前版本不同的传感器处理方式。一般来说,新旧不兼容。但是也有少许例外。lejos.robotics中有一些转换器adaptor提供兼容。如果你不打算大幅修改你现有的代码,我们建议你在这个package中找一找有没有adaptor能够兼容。与filter类似,adaptor也可以复合使用。如果你没有找到合适的adaptor,那么package中现有的adaptor是一个很好的例子,你可以通过这些例子自己写一个adaptor
(呼~终于翻译完了,好累好累 才疏学浅,各位轻拍~)
|
|